One of the biggest benefits of GraphQL is the built-in type-system. These types can then be used when building your frontend to make sure you have end-to-end type safety.
Instead of writing TypeScript types manually to match your Grafbase GraphQL API, we can use GraphQL Code Generator to automatically generate everything we need.
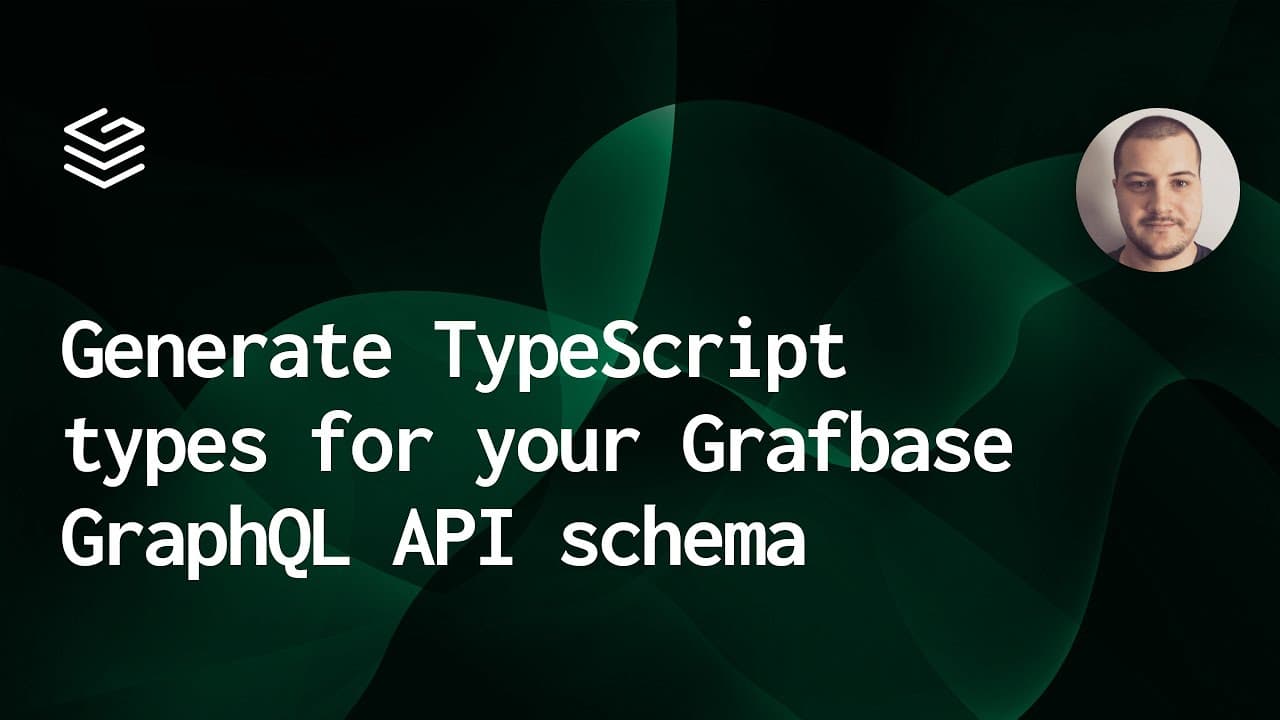
Install the following dependencies to get started:
typescript
@graphql-codegen/cli
@graphql-codegen/client-preset
npm install -D typescript @graphql-codegen/cli @graphql-codegen/client-preset
Then inside package.json
add to scripts
a new command:
{
"codegen": "graphql-codegen -r dotenv/config"
}
You'll notice above we're preloading dotenv/config
which means we can use environment variables instead of committing our Grafbase API URL and API Key to source control.
Create .env
in the root of your project and add your project API URL and API Key:
GRAFBASE_API_URL=
GRAFBASE_API_KEY=
Next inside of the project root, create the file codegen.ts
and add your Grafbase API URL and API key:
import { CodegenConfig } from '@graphql-codegen/cli'
const config: CodegenConfig = {
schema: {
[process.env.GRAFBASE_API_URL]: {
headers: {
'x-api-key': process.env.GRAFBASE_API_KEY,
},
},
},
}
export default config
Now we've configured the location of the schema we can configure the files we want to generate, and the plugins we want to use.
We'll be using the client-preset
to generate the folder gql
in the root of our project. You can update this to output where you like.
import { CodegenConfig } from '@graphql-codegen/cli'
const config: CodegenConfig = {
schema: {
[process.env.GRAFBASE_API_URL]: {
headers: {
'x-api-key': process.env.GRAFBASE_API_KEY,
},
},
},
generates: {
'./gql/': {
preset: 'client',
plugins: [],
},
},
}
export default config
Finally, we'll want to ignore the warnings for no documents:
import { CodegenConfig } from '@graphql-codegen/cli'
const config: CodegenConfig = {
schema: {
[process.env.GRAFBASE_API_URL]: {
headers: {
'x-api-key': process.env.GRAFBASE_API_KEY,
},
},
},
generates: {
'./gql/': {
preset: 'client',
plugins: [],
},
},
ignoreNoDocuments: true,
}
export default config
That's it!
We can now run the script codegen
to generate the TypeScript types for the provided Grafbase API schema:
npm run codegen
You should now have the folder gql
in the root of your project (or where you set it) that contains the generated TypeScript types for all scalars, queries, mutations, types, input types, enumerations, and more.
Here's what an example schema, codegen config, and generated TS looks like:
# grafbase/schema.graphql
type Post @model {
title: String
author: Author!
published: Boolean!
}
type Author @model {
name: String
posts: [Post!]!
}
Because we're using the client-preset
with GraphQL Code Generator we can automatically generate types for graphql operations — learn more.